StringLastIndexOf
Searches a string for the last occurrence of a specified sub-string.
Syntax
StringLastIndexOf( StringToSearch, SubstringToFind, SearchStartIndex, SearchLength );
Parameters
Parameter Name | Type | Description | Optional? | Default Value |
---|---|---|---|---|
StringToSearch | String | The string to search. | No | n/a |
SubstringToFind | String | The sub-string you want to find inside StringToSearch. | No | n/a |
SearchStartIndex | Integer | The index location in StringToSearch where the function should start the search. If left as an empty string, the search will start from the first character. | Yes | "" |
SearchLength | Integer | The number of characters to include in the search, including the starting character. If left as an empty string, the full string will be searched. | Yes | "" |
Output
If one instance of the specified sub-string is found, the function will return the index number of the first character of the sub-string's last occurrence; otherwise, it will return a value of -1.
Use Case
Consider the following example string, which contains three instances of the word "bread":
"brown bread white bread pitta bread"
If you used the StringLastIndexOf function to find the sub-string "bread" without using any of the optional parameters, the result would be the index of the letter "b" found in "pitta bread".
StringLastIndexOf( "brown bread white bread pitta bread", "bread" );
= 30
We now know this instance of the word "bread" begins at index 30 and - because it has five characters - ends at index 34. As a result, if you include a SearchStartIndex argument lower than 34 with the above example, the instance of "bread" found using default arguments will not be found, because every character after SearchStartIndex is ignored.
If you use 32 as your SearchStartIndex, the function will effectively be looking at the following string:
"brown bread white bread pitta bre"
In other words, if we use the following function:
StringLastIndexOf( "brown bread white bread pitta bread", "bread", 32 );
= 18
This time, the instance of "bread" in "pitta bread" is not found. Instead, the preceding instance in "white bread" is found, at index 18.
Adding an argument for SearchLength will restrict the length of the string being examined. This is useful when examining larger strings if you want to limit your search to within a certain number of characters, avoiding unnecessary processing time.
In the bread example, we know that as long as the whole string contains at least one instance of "bread", an index will be returned as long as the search string length is long enough to encompass one instance. If you look at the diagram below, you will see that as long as your search string is at least 16 characters in length, you will return at least one instance of the word "bread", and an index as a result.
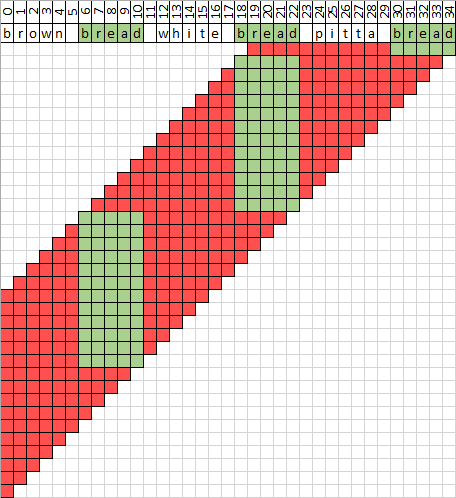
All search strings containing 16 or more characters will return at least one instance of the word "bread".
Conversely, if our search string length is less than the total length of the string being searched for, it will never find the whole word "bread", no matter what other arguments are used.
StringLastIndexOf( "brown bread white bread pitta bread", "bread", 34, 4 );
= -1